엘라의 개발 스케치 Note
[CS 전공지식 노트] 1.1.2 팩토리 패턴 본문
# 팩토리 패턴
더보기
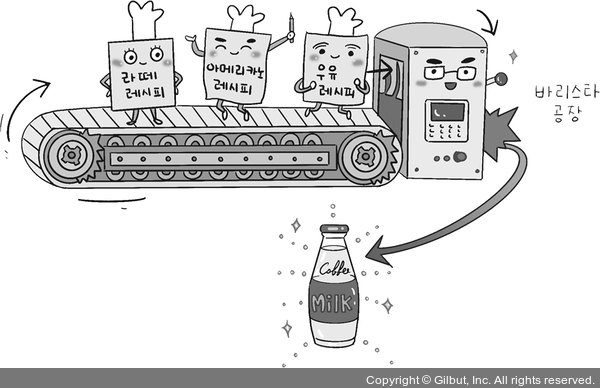
? 팩토리 패턴
- 객체를 사용하는 코드에서 객체 생성 부분을 떼어내 추상화한 패턴이자
상속 관계에 있는 두 클래스에서 상위 클래스가 중요한 뼈대를 결정하고,
하위 클래스에서 객체 생성에 관한 구체적인 내용을 결정하는 패턴
! 장점과 예시
- 장점
- 상하위 클래스 분리 -> 느슨한 결합
- 상위 클래스 인스턴스 생성 방식 무지 -> 더 많은 유연성
- 객체 생성 로직 분리 -> 유지 보수성 증가
- 예시
- (하위) 각각 레시피 -> (상위) 바리스타 공장 => 생산
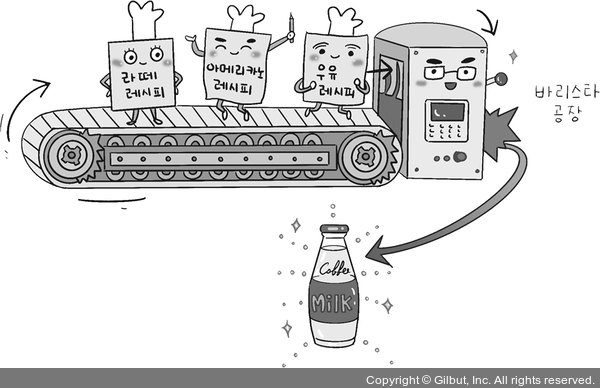
! 자바스크립트의 팩토리 패턴
더보기
- new Object()로 구현
- 전달 받은 값에 따라 다른 타입의 객체를 생성하며 인스턴스의 타입 등을 정함
const num = new Object(42)
const str = new Object('abc')
num.constructor.name; // Number
str.constructor.name; // String
- 커피 팩토리 기반 라떼 등을 생산하는 코드
- (상위) CoffeeFactory 중요한 뼈대 결정 / (하위) LatteFactory 구체적인 내용 결정
- cf. 의존성 주입 (LatteFactorty 생성 인스턴스를 CoffeeFactory에 주입)
- static 정적 메소드 정의 -> 클래스 기반 객체를 만들지 않고 호출 가능, 해당 메서드에 대한 메모리를 한 번만 할당할 수 있다는 장점
- (상위) CoffeeFactory 중요한 뼈대 결정 / (하위) LatteFactory 구체적인 내용 결정
class CoffeeFactory {
static createCoffee(type) {
const factory = factoryList[type]
return factory.createCoffee()
}
}
class Latte {
constructor() {
this.name = "latte"
}
}
class Espresso {
constructor() {
this.name = "Espresso"
}
}
class LatteFactory extends CoffeeFactory{
static createCoffee() {
return new Latte()
}
}
class EspressoFactory extends CoffeeFactory{
static createCoffee() {
return new Espresso()
}
}
const factoryList = { LatteFactory, EspressoFactory }
const main = () => {
// 라떼 커피를 주문한다.
const coffee = CoffeeFactory.createCoffee("LatteFactory")
// 커피 이름을 부른다.
console.log(coffee.name) // latte
}
main()
! 자바의 팩토리 패턴
더보기
- if ("Latte".equalsIgnoreCase(type)) 문자열 비교 기반 로직 => Enum 또는 Map을 이용한 매핑도 가능
- Enum: 상수의 집합을 정의할 때 사용되는 타입
- Java에서 다른 언어보다 더 활발히 활용됨
- 메스드를 집어넣어 관리할 수도 있음
- 코드 리팩터링 시 상수 집합에 대한 로직 수정 시 이 부분만 수정하면 된다는 장점
- 스레드 세이프 -> 싱글톤 패턴 시 도움
- Enum: 상수의 집합을 정의할 때 사용되는 타입
enum CoffeeType {
LATTE,
ESPRESSO
}
abstract class Coffee {
protected String name;
public String getName() {
return name;
}
}
class Latte extends Coffee {
public Latte() {
name = "latte";
}
}
class Espresso extends Coffee {
public Espresso() {
name = "Espresso";
}
}
class CoffeeFactory {
public static Coffee createCoffee(CoffeeType type) {
switch (type) {
case LATTE:
return new Latte();
case ESPRESSO:
return new Espresso();
default:
throw new IllegalArgumentException("Invalid coffee type: " + type);
}
}
}
public class Main {
public static void main(String[] args) {
Coffee coffee = CoffeeFactory.createCoffee(CoffeeType.LATTE);
System.out.println(coffee.getName()); // latte
}
}
'CS > 면접을 위한 CS 전공지식 노트' 카테고리의 다른 글
[CS 전공지식 노트] 1.1.4 옵저버 패턴 (0) | 2024.03.08 |
---|---|
[CS 전공지식 노트] 1.1.3 전략 패턴 (0) | 2024.03.06 |
[CS 전공지식 노트] 1.1.1 싱글톤 패턴 (0) | 2024.03.04 |
[CS 전공지식 노트] 1.1 디자인 패턴 (0) | 2024.03.04 |
[CS 전공지식 노트] 1장. 디자인 패턴과 프로그래밍 패러다임 (0) | 2024.03.04 |
Comments